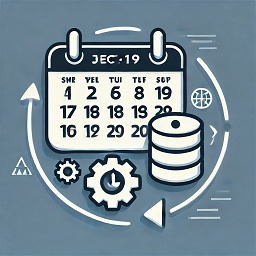
Using Cron to schedule a backup of important files
To have your script run once every day, you can use cron, which is a Linux utility for scheduling tasks. Here’s how you can set it up:
Steps to Schedule the Script with Cron:
-
Place your script in a directory:
Ensure that thecreate_backup.sh
script is saved somewhere on your server, for example, in/srv/scripts/create_backup.sh
. - Make the script executable:
Make sure the script has the appropriate executable permissions:chmod +x /srv/scripts/create_backup.sh
- Edit the root user’s crontab:
Since the script needs to run as root, you will need to add it to the root user’s crontab. You can do this by running:sudo crontab -e
-
Add a cron job to run the script daily:
Add the following line to the root’s crontab file to schedule the script to run every day at, for example, 2:00 AM:0 2 * * * /srv/scripts/create_backup.sh
Explanation of the cron syntax:
0 2 * * *
means “at 2:00 AM every day.”/srv/scripts/create_backup.sh
is the full path to the script.
If you want it to run at a different time, you can modify the time accordingly:
- First value: minute (0-59)
- Second value: hour (0-23)
- Third value: day of the month (1-31)
- Fourth value: month (1-12)
- Fifth value: day of the week (0-7, where both 0 and 7 are Sunday)
- Save and Exit:
After adding the line, save and exit the crontab editor (in most editors,Ctrl + O
to save andCtrl + X
to exit).
Verifying the Cron Job:
- To verify that the cron job has been added, you can list the cron jobs with:
sudo crontab -l
Now, the create_backup.sh
script will run automatically every day at 2:00 AM (or your chosen time).
Log Output:
If you want to log the script’s output to a file for troubleshooting or review, you can modify the cron job to include a redirection to a log file:
0 2 * * * /srv/scripts/create_backup.sh >> /var/log/backup_script-config.log 2>&1
This will append both standard output and error messages to /var/log/backup_script.log
.
Backup Script
My backup script create a package with the files and directories listed and save it in a directory that is duplicated
#!/bin/bash
# ┌────────────────────────────────────────────────────────────────────────────────┐
# │ │
# │ create_backup.sh │
# │ a script that will create a package with the files and directories below │
# │ needs to be run as root. It will create a package to restore the files later │
# ┼────────────────────────────────────────────────────────────────────────────────┼
# │ Guillaume Plante <guillaumeplante.qc@gmail.com> │
# └────────────────────────────────────────────────────────────────────────────────┘
# Colors for output
UL='\033[4;93m'
IL='\033[3;91m'
BL='\033[6;94m'
CYAN='\033[0;36m'
YELLOW='\033[0;33m'
RED='\033[0;31m'
NC='\033[0m' # No Color
LogCategory=backup
# handy logging and error handling functions
pecho() { printf %s\\n "$*"; }
log() { pecho "$@"; }
# Verbose output function
log_info() {
echo -e "${CYAN}[INFO] $1${NC}"
logger --tag $LogCategory -p user.info "[INFO] $1"
}
log_il() {
echo -e "${IL}$1${NC}"
logger --tag $LogCategory -p user.info "$1"
}
log_warning() {
echo -e "${YELLOW}[WARNING] $1${NC}"
logger --tag $LogCategory -p user.warning "[WARNING] $1"
}
log_error() {
echo -e "${RED}[ERROR] $1${NC}"
logger --tag $LogCategory -p user.error "[ERROR] $1"
}
debug() { : log_info "INFO: $@" >&2; }
error() { log_info "ERROR: $@" >&2; }
fatal() { log_error "$@"; exit 1; }
try() { "$@" || fatal "'$@' failed"; }
usage_fatal() { usage >&2; pecho "" >&2; fatal "$@"; }
log_il "\ncreate_backup.sh - creating a backup of my scripts\n"
# Ensure the script is run as root
if [ "$EUID" -ne 0 ]; then
log_error "Please run as root"
exit 1
fi
# Directory to store backups
backup_dir="/srv/backup/scripts-config"
# Create backup directory if it doesn't exist
if [ ! -d "$backup_dir" ]; then
mkdir -p "$backup_dir"
fi
# Set the output filename for the backup tarball
backup_file="$backup_dir/backup_scripts-config_$(date +%Y%m%d).tar.gz"
# List of files and directories to include in the package
files_to_backup=(
"/root/.ssh"
"/etc/samba/smb.conf"
"/etc/initramfs-tools/initramfs.conf"
"/etc/initramfs-tools/root/.ssh"
"/home/gp/.ssh"
"/home/gp/scripts"
"/srv/secrets"
"/srv/scripts"
"/srv/vpn/config"
"/srv/vpn/scripts"
"/home/storage/Configs/QBittorrentVPN/openvpn"
"/home/storage/Configs/QBittorrentVPN/qBittorrent/config"
"/etc/dropbear"
)
# Excluded directories
excluded_dirs=(
"--exclude=/srv/vpn/config/vpn-configs-contrib"
"--exclude=/srv/vpn/config/transmission-home"
)
# Create the tarball, with verbose output (-v), preserving file permissions (-p), and excluding specified directories
log_info "Creating backup package..."
tar -cpvzf $backup_file "${excluded_dirs[@]}" --absolute-names "${files_to_backup[@]}"
# Check if the tarball was created successfully
if [ $? -eq 0 ]; then
log_info "Backup successfully created at: $backup_file"
else
log_error "An error occurred while creating the backup."
exit 1
fi
# Step to delete backups older than 10 days (log rotation)
log_info "Performing log rotation, deleting backups older than 10 days..."
find $backup_dir -type f -name "backup_*.tar.gz" -mtime +10 -exec rm -f {} \;
log_info "Backup creation and log rotation complete."