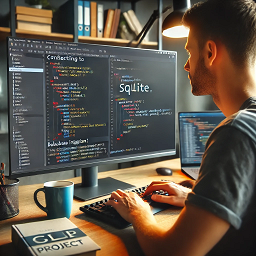
How to Get System.Data.SQLite
and SQLite.Interop.dll
by Creating a .NET C# Project
If you are working on a project that involves SQLite, you may need both System.Data.SQLite
and SQLite.Interop.dll
files. This guide will walk you through obtaining these DLLs by creating a .NET C# project with a dependency on SQLite. By creating a .NET C# project and adding the System.Data.SQLite
dependency, you can easily obtain both System.Data.SQLite.dll
and SQLite.Interop.dll
. Moreover, modifying the project file gives you control over how these DLLs are managed during the build process. This approach ensures a smooth experience when using SQLite in your applications, especially when managing deployment scenarios.
Step 1: Create a New C# Project
To get started, create a new .NET C# project. You can do this using Visual Studio or the .NET CLI. Below are the instructions for both methods.
Using Visual Studio
- Open Visual Studio.
- Go to File > New > Project.
- Select Console App (.NET Core) or Console App (.NET Framework) based on your requirements, and click Next.
- Name your project (e.g.,
SQLiteDemo
) and click Create.
Using the .NET CLI
If you prefer the command line, open your terminal and run:
dotnet new console -n SQLiteDemo
This will create a new console application named SQLiteDemo
.
Step 2: Add the SQLite Dependency
To use SQLite in your C# project, add the System.Data.SQLite
package. You can do this via NuGet in Visual Studio or by using the .NET CLI.
Using Visual Studio
- Right-click on your project in the Solution Explorer.
- Select Manage NuGet Packages.
- Search for
System.Data.SQLite
and click Install.
Using the .NET CLI
Run the following command in your terminal:
cd SQLiteDemo
dotnet add package System.Data.SQLite
This will add the necessary SQLite package to your project.
Step 3: Modify the Project File
In order to ensure that SQLite.Interop.dll
is correctly managed during the build process, you need to modify the project file (.csproj
) to include some specific properties. These properties control how the interop DLL is included and managed in the output directory.
Edit the Project File
- Open your
.csproj
file in a text editor or within Visual Studio. -
Add the following properties inside the
<PropertyGroup>
section:<ContentSQLiteInteropFiles>true</ContentSQLiteInteropFiles> <CopySQLiteInteropFiles>false</CopySQLiteInteropFiles> <CleanSQLiteInteropFiles>false</CleanSQLiteInteropFiles> <CollectSQLiteInteropFiles>false</CollectSQLiteInteropFiles>
These properties do the following:
<ContentSQLiteInteropFiles>true</ContentSQLiteInteropFiles>
: Ensures that theSQLite.Interop.dll
file is included as a content file.<CopySQLiteInteropFiles>false</CopySQLiteInteropFiles>
: Prevents automatic copying of theSQLite.Interop.dll
file to the output directory.<CleanSQLiteInteropFiles>false</CleanSQLiteInteropFiles>
: Prevents the deletion of the interop files during the clean process.<CollectSQLiteInteropFiles>false</CollectSQLiteInteropFiles>
: Prevents the collection of interop files in certain build scenarios.
This configuration ensures that you have more control over how SQLite.Interop.dll
is managed, especially if you have specific requirements for deployment or packaging.
Step 4: Restore and Build the Project
Once the dependency is added and the project file is updated, restore and build the project.
Using Visual Studio
Visual Studio should automatically restore the project after adding the package and modifying the .csproj
file. If it doesn’t, right-click on your solution and select Restore NuGet Packages.
To build the solution, click Build > Build Solution.
Using the .NET CLI
Run the following commands:
dotnet restore
dotnet build
Step 5: Locate the DLLs
After building the project, you will find both System.Data.SQLite.dll
and SQLite.Interop.dll
in your project’s output directory.
-
Output Directory: The DLLs will be located in the
bin
directory, typically at:<Project Directory>/bin/Debug/net<version>/
For example, if you created a .NET 6 project, the path would be
bin/Debug/net6.0/
.
Step 6: Verify SQLite Usage in Your Code
To verify that everything works, add a simple code snippet that interacts with SQLite:
using System;
using System.Data.SQLite;
namespace SQLiteDemo
{
class Program
{
static void Main(string[] args)
{
string connectionString = "Data Source=:memory:;Version=3;";
using (var connection = new SQLiteConnection(connectionString))
{
connection.Open();
Console.WriteLine("SQLite connection established successfully!");
// Your code to interact with SQLite database goes here
}
}
}
}
Or in PowerShell
function Add-SqlLiteTypes {
# Load the SQLite assembly
[string[]]$AllAssemblies = "SQLite.Interop.dll", "System.Data.SQLite.dll"
ForEach($assembly in $AllAssemblies){
$assemblyPath = Join-Path -Path (Get-ScriptsPath) -ChildPath $assembly
try{
Add-Type -Path "$assemblyPath" -ErrorAction Stop
Write-Host "SQLite assembly $assembly loaded successfully."
}catch{
Write-Warning "Failed to load SQLite assembly $assembly : $_"
}
}
}
# Function to load SQLite database
function Load-SQLiteDatabase {
param (
[string]$DatabasePath
)
Write-Host "Attempting to open SQLite database at $DatabasePath..." -ForegroundColor DarkCyan
# SQLite connection string
$connectionString = "Data Source=$DatabasePath;Version=3;"
# Create SQLite connection
$connection = New-Object -TypeName System.Data.SQLite.SQLiteConnection -ArgumentList $connectionString
$connection.Open()
Write-Host "Successfully connected to SQLite database." -ForegroundColor DarkCyan
return $connection
}
# Function to insert a URI record into the SQLite database, including the Version
function Insert-UriIntoDatabase {
param (
[System.Data.SQLite.SQLiteConnection]$Connection,
[PSCustomObject]$UriObject,
[int]$Version
)
$dateAdded = [DateTime]::Now
$query = @"
INSERT INTO magnet_links (DateAdded, AbsolutePath, AbsoluteUri, Authority, Host, Port, Scheme, Version)
VALUES (@DateAdded, @AbsolutePath, @AbsoluteUri, @Authority, @Host, @Port, @Scheme, @Version);
"@
$command = $Connection.CreateCommand()
$command.CommandText = $query
# Add parameters to prevent SQL injection
$command.Parameters.Add([System.Data.SQLite.SQLiteParameter]::new("@DateAdded", $dateAdded))
$command.Parameters.Add([System.Data.SQLite.SQLiteParameter]::new("@AbsolutePath", $UriObject.AbsolutePath))
$command.Parameters.Add([System.Data.SQLite.SQLiteParameter]::new("@AbsoluteUri", $UriObject.AbsoluteUri))
$command.Parameters.Add([System.Data.SQLite.SQLiteParameter]::new("@Authority", $UriObject.Authority))
$command.Parameters.Add([System.Data.SQLite.SQLiteParameter]::new("@Host", $UriObject.Host))
$command.Parameters.Add([System.Data.SQLite.SQLiteParameter]::new("@Port", $UriObject.Port))
$command.Parameters.Add([System.Data.SQLite.SQLiteParameter]::new("@Scheme", $UriObject.Scheme))
$command.Parameters.Add([System.Data.SQLite.SQLiteParameter]::new("@Version", $Version))
# Execute the insert command
$command.ExecuteNonQuery() | Out-Null
Write-Host "Inserted URI: $($UriObject.AbsoluteUri) with Version: $Version into the database." -ForegroundColor DarkCyan
}
Run the project to ensure the SQLite connection is established without any errors.