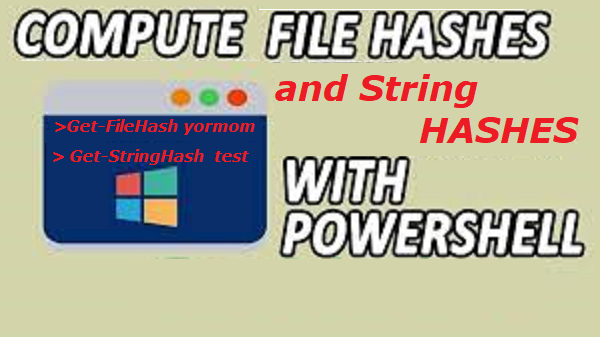
Validating File Integrity with Get-FileHash
PowerShell provides a cmdlet to computes the hash value for a file by using a specified hash algorithm. Get-FileHash
.
What is a hash?
A hash is a string of characters that is generated by analyzing the bytes of the file using a specific algorithm. This hash value is much smaller than the actual size of the file and is published alongside the file you’re downloading, which allows you to run that same hash algorithm against your downloaded file and verify the hashes match.
There are different algorithms and utilities to generate these hash values. Every algorithm will generate a different hash, but the utility used to generate the hash will always generate the same hash value when you choose the same algorithm.
Powershell cmdlet
Previously you needed a 3rd party tool to do this, but PowerShell provides a handy cmdlet to perform the computations for you. Get-FileHash
is the built-in PowerShell cmdlet that can be used to generate a hash value, allowing you to verify against the reference hash. Find more details on the cmdlet and options here
> Get-FileHash -Path .\cp045967.exe
Algorithm Hash Path
--------- ---- ----
SHA256 82F776A89483EB2192FC41637708A820938B9091D5964530A089092CB64AEBFB C:\Down\Blog\cp045967.exe
You’ll see it generated a hash value of 82F776A89483EB2192FC41637708A820938B9091D5964530A089092CB64AEBFB, and you can compare that to the value on the web page and verify it matches.
You can check multiple files, too. If you have downloaded all three files on that page, you can use a wildcard in the path and get them all.
> Get-FileHash -Path .\*.*
Algorithm Hash Path
--------- ---- ----
SHA256 82F776A89483EB2192FC41637708A820938B9091D5964530A089092CB64AEBFB C:\Down\Blog\cp045967.exe
SHA256 71EF16D38226ED06E72B1A87493AA90521D62D18DCF68BB15014C3C1028FBF4C C:\Down\Blog\cp045967_part1.compsig
SHA256 8B6A297F69E570D72111C076505BFC074AB84B618B9142129CC8746525DE49F6 C:\Down\Blog\cp045967_part2.compsig
Important Notes
Here are a few items to be aware of:
- The larger the file, the longer it takes to compute the hash. For example, 500GB images can take a couple of hours.
- The hash is based on the contents, not the date/time stamps of the file, etc.
- For example, if you create a file called “HelloWorld.txt” and put “Hello World” in it, the hash is:
A591A6D40BF420404A011733CFB7B190D62C65BF0BCDA32B57B277D9AD9F146E
- If you rename the file to “GoodbyeWorld.txt”, the hash remains the same.
- If you change the text inside the file to “Goodbye World” and save it, the hash is now:
C96724127AF2D6F56BBC3898632B101167242F02519A99E5AB3F1CAB9FF995E7
- And if I change the text inside the file back to “Hello World”, the hash is back to the original value.
- You can generate a file and put the same text in your file, and get the same hash value, as noted above. Hashes are computed based on the contents to ensure their integrity. If you change the “Hello World” to “Hello world” (lowercase w on “world), you’ll see that you get a completely different hash.
Small PowerShell Script to get a string hash
For some cases, you want to calculate the hash of a string, not the content of a file. We can still leverage Get-FileHash
this way for this purpose, using the InputStream
argument:
function Get-StringHash{
<#
.SYNOPSIS
Computes the hash value for a string
.DESCRIPTION
Computes the hash value for a string
#>
[CmdletBinding(SupportsShouldProcess)]
Param
(
[Parameter(Mandatory = $true, Position = 0)]
[ValidateNotNullOrEmpty()]
[string]$String,
[Parameter(Mandatory = $false)]
[ValidateSet("MD5","SHA1","SHA256","SHA384", "SHA512")]
[string]$Algorithm = "SHA256"
)
try{
$Result = Get-FileHash -Algorithm $Algorithm -InputStream ([IO.MemoryStream]::new([byte[]]([char[]]"$String")))
return $Result.Hash
}catch{
Write-Error "$_"
}
}
Results
> Get-StringHash -String "test"
9F86D081884C7D659A2FEAA0C55AD015A3BF4F1B2B0B822CD15D6C15B0F00A08